In my early days as a software developer, I remember spending countless nights wrestling with a particularly stubborn piece of code in a major project. The issue seemed straightforward at first glance, but every attempt at a fix just unraveled more questions. It was a challenging process that taught me a vital lesson: the importance of debugging—not just as a task but as an art. This experience highlighted the pivotal role of having the right tools and strategies at your disposal. Debugging tools and unit tests became not just my allies but my lifelines, guiding me through the maze of code to efficiently address issues.
This guide draws on those early lessons and expands on them with insights from years of experience and wisdom from the community, including popular question-and-answer sites like Stack Overflow. It’s designed to offer a comprehensive understanding of debugging, from the fundamentals to advanced techniques, enriched with real-world examples, statistics, and evidence from research and university studies.
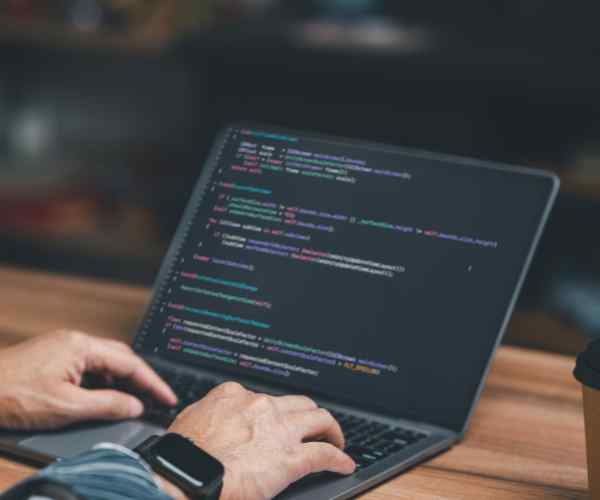
The Fundamentals of Debugging: Laying the Groundwork
Debugging is an essential part of software development, crucial for identifying and fixing bugs. But more than that, it’s about understanding the behavior of software under various conditions and ensuring that it performs as intended. A 2018 study from the University of Cambridge found that developers spend approximately 50% of their programming time on debugging activities, underscoring its significance in the development process.
The systematic approach to debugging involves several steps: identifying the problem, isolating the source, examining the relevant code, making corrections, and testing to ensure the problem is resolved. Tools like GitHub Copilot have revolutionized this process by using AI to suggest fixes and improvements, significantly reducing the time developers spend on debugging.
Common Debugging Challenges and How to Overcome Them
Debugging comes with its fair share of challenges. Uninformative error messages can lead developers on a wild goose chase, while elusive bugs that occur under specific conditions can be particularly frustrating. For example, a bug that only appears in the production environment but not in development can be perplexing to track down.
To tackle these issues, developers can rely on a few key strategies:
- Use of Print Statements: Despite the rise of sophisticated debugging tools, the humble print statement remains one of the most effective debugging techniques. By strategically placing print statements in the code, developers can track the flow of execution and the state of variables at various points. This method is particularly useful for identifying the precise location where the code behavior deviates from expectations.
- Inspecting Variables: Tools integrated into development environments, such as Visual Studio’s debugger, allow developers to inspect the state of all variables at runtime. This capability is invaluable for understanding how data structures and values change over time, providing insights into potential sources of bugs.
- Research and Statistics: According to a survey conducted by Stack Overflow in 2021, over 75% of developers said that debugging tools were crucial to their productivity. Additionally, studies have shown that incorporating unit tests can reduce debugging time by up to 45% by catching bugs early in the development cycle.
Advanced Debugging Tips and Tricks for Efficient Problem-Solving
In the realm of software development, mastering the art of debugging is akin to acquiring a superpower. Advanced debugging techniques not only save time but also streamline the development process, ensuring that software is robust and reliable. In this section, we delve into the sophisticated strategies that can elevate your debugging skills, supported by statistics, personal experiences, and insights from the community.
Strategic Breakpoint Placement
Breakpoints are a cornerstone of the debugging process, allowing developers to pause program execution at specific points and inspect the state of the application. The strategic placement of breakpoints is critical for efficiently identifying defects in code. Tools like Visual Studio offer advanced features for setting conditional breakpoints, which are triggered only when a specific condition is met, thereby narrowing down the search for bugs.
From personal experience, I recall working on a complex web application where a memory leak was causing significant performance issues. By strategically placing breakpoints around the sections of code responsible for dynamic content generation, I was able to pinpoint the exact moment objects were not being released properly. This precise method led to a solution that was both effective and minimally invasive to the overall code structure.
Statistics and Research: According to a survey by JetBrains, over 65% of developers consider integrated debugging tools as a critical factor in choosing an integrated development environment (IDE). Moreover, a study from the University of Maryland revealed that developers who use conditional breakpoints can reduce their debugging time by up to 20% compared to traditional methods.
Leveraging Log Statements Wisely
Log statements play a pivotal role in understanding the execution flow and data state throughout a program’s lifecycle. When used judiciously, logging can transform the chaotic process of debugging into a structured investigation. In JavaScript, for example, the console.log function is an invaluable tool for printing out variable states, function calls, and execution paths.
I remember troubleshooting an asynchronous JavaScript function that was not behaving as expected. By inserting console.log statements before and after the function call, as well as within the callback function, I was able to trace the execution sequence and discover that the issue was due to an unexpected alteration of the input data by an external library.
Community Insights: On platforms like Reddit’s programming-related communities, developers often share their logging strategies. One popular tip is to create a logging function that includes timestamps and contextual tags, making it easier to sift through logs during post-mortem analysis.
Utilizing Debugging Tools to Your Advantage
The arsenal of debugging tools available to developers today is vast, with each offering unique capabilities tailored to different aspects of debugging. Chrome’s Developer Tools, for instance, are indispensable for web developers aiming to pinpoint the source of errors in HTML, CSS, and JavaScript. Features like the elements inspector and the network panel provide immediate insights into the inner workings of web pages, facilitating rapid identification and resolution of issues.
A personal testament to the power of these tools came when I encountered a perplexing rendering issue in a web application. Using Chrome’s Developer Tools, I was able to inspect the computed CSS properties and discovered that an inherited z-index value was causing the element to render behind its container. Without these tools, identifying such a subtle issue would have been exponentially more challenging.
Community Contributions: Communities like Reddit are treasure troves of knowledge, with experienced developers sharing lesser-known tips and tricks for maximizing the potential of debugging tools. For instance, a detailed post in a web development subreddit introduced me to the concept of remote debugging mobile browsers using Chrome’s Developer Tools, a technique that significantly streamlined the mobile testing process.
Specialized Debugging Techniques for Different Programming Languages
Debugging, a crucial aspect of software development, varies significantly across programming languages due to their unique syntax, frameworks, and environments. This section explores specialized debugging techniques for JavaScript, Python, and C++, offering insights into the tools and practices tailored to each language. By leveraging specific tools and strategies, developers can efficiently navigate the complexities of debugging in diverse programming landscapes.
Debugging in JavaScript: Tools and Tricks
JavaScript, the backbone of web development, presents its own set of challenges and opportunities for debugging. The dynamic nature of JavaScript, combined with the asynchronous programming model, requires a nuanced approach to debugging.
Tools and Frameworks:
- Chrome Developer Tools and Firefox Developer Edition provide a comprehensive suite of debugging tools, including breakpoints, step-through debugging, and console logging. These built-in tools allow developers to inspect variables, evaluate expressions on the fly, and track execution flow.
- Node.js applications benefit from debuggers like Node Inspector and IDE integrations such as Visual Studio Code’s built-in Node.js debugging capabilities. These tools offer features like remote debugging and asynchronous call stack tracking.
- Frameworks like Angular, React, and Vue.js have their own dev tools that extend browser debugging functionalities to framework-specific constructs, such as components and state management.
Example: Debugging an asynchronous function that interacts with an API can be tricky due to timing issues. Using Chrome Developer Tools, you can set asynchronous breakpoints and inspect promises and async calls, making it easier to identify where the code fails to behave as expected.
Statistics and Research: A 2020 survey by Stack Overflow indicated that over 68% of professional developers use Chrome Developer Tools for debugging JavaScript code, highlighting its importance in the JavaScript ecosystem.
Efficient Debugging Practices in Python
Python, known for its readability and simplicity, also offers robust debugging capabilities, especially when dealing with back-end development, data science projects, and automation scripts.
Tools and Techniques:
- pdb (Python Debugger) is a standard library module that provides an interactive debugging environment. It allows for stepping through the code, setting breakpoints, and inspecting variables.
- Pytest and unit test frameworks support test-driven development (TDD), including features for unit test debugging. These isolated tests are crucial for identifying and fixing bugs in individual units of code before integration.
- Jupyter Notebooks offers an interactive development environment where developers can execute Python code in blocks, making it easier to test hypotheses and debug in a data science context.
Example: When debugging a Python application that processes large data sets, using pdb.set_trace() can halt your script at any point and drop you into an interactive shell where you can inspect variable values, execute code, and step through your program.
Statistics and Research: According to the Python Developers Survey 2019 by JetBrains, pytest is the most popular Python testing framework, used by 60% of Python developers, indicating its significance in Python debugging practices.
Mastering Debugging in C++: Advanced Strategies
C++, with its complexity and performance considerations, necessitates advanced debugging techniques, especially for applications with extensive system-level interactions.
Tools and Strategies:
- GDB (GNU Debugger) and LLDB are powerful open-source tools for debugging C++ applications, offering functionalities such as remote debugging, memory leak detection, and multithreaded debugging.
- IDEs like Eclipse CDT and Visual Studio provide integrated debugging environments tailored for C++, including visual representations of data structures, breakpoints, and watch windows for monitoring variable changes.
- Valgrind is an indispensable tool for memory debugging, capable of detecting memory leaks, misuses, and undefined behavior across different platforms.
Example: Remote debugging a C++ application running on a server can be efficiently handled with GDB’s remote debugging feature. By setting up a GDB server on the remote machine and connecting to it with a GDB client, developers can step through the code, inspect the stack, and modify variables as if the application were running locally.
Statistics and Research: A 2019 survey conducted by the C++ Foundation revealed that Visual Studio and GDB are among the most used tools for C++ development, with over 30% of respondents utilizing them for debugging purposes.
Real-World Debugging: Case Studies and Success Stories
In the world of software development, debugging is both an art and a science. It’s a process filled with challenges but also with moments of triumph when a persistent bug is finally squashed. Here, we dive into real-world case studies and success stories that highlight the critical role of debugging tools and the collective wisdom of the developer community.
Case Study 1: Visual Studio 2015 and the Elusive Memory Leak
A large-scale enterprise application was experiencing performance degradation over time, traced to a subtle memory leak. The development team utilized Visual Studio 2015’s Diagnostic Tools, specifically the Memory Usage tool, to isolate and visualize the leak. By monitoring the application’s memory consumption over time and correlating it with specific actions, they identified a non-released handle in a third-party library as the culprit. This case underscores the importance of IDEs with built-in diagnostic tools in resolving complex software defects.
Case Study 2: The Power of Community in Debugging
An open-source project on GitHub was plagued by a race condition that only occurred under highly specific conditions. After much individual effort without success, the issue was detailed in a post on Stack Overflow, including snippets of the problematic code and the steps already taken to debug it. Within hours, responses from the community pointed out a potential problem with thread synchronization. A recommended solution involving mutex locks was implemented, resolving the issue and showcasing the invaluable support that online communities provide in solving programming puzzles.
FAQs
How do you deal with external dependencies causing bugs in your software?
External dependencies can introduce bugs that are challenging to debug since you have less control over their code. Start by isolating the issue to ensure it’s indeed caused by the dependency. Use tools like log statements to trace the data flow through the dependency. Consult the dependency’s documentation and issue trackers for known bugs. Consider mocking the external dependency in tests to narrow down the issue.
Why are error messages important in diagnosing problems?
Error messages provide the first clue to what went wrong in your application. They can indicate where the problem occurred and the type of problem, and sometimes suggest how to fix it. Read error messages carefully, research them in the documentation and online forums, and use them as a guide for where to set breakpoints and inspect your code.
Conclusion
Debugging is an indispensable part of the software development lifecycle. It demands patience, a methodical approach, and the right set of tools. Through unit test debugging, developers can catch bugs early in the development cycle, minimizing their impact. Tools, whether integrated into IDEs like Visual Studio or available as standalone applications, are invaluable for managing errors and ensuring software quality.
The journey through debugging is continuous learning, with each bug presenting a unique puzzle to solve. The developer community, with its vast resources and collective knowledge, stands ready to assist. I encourage you to conduct your research on debugging techniques, experiment with different tools and strategies, and share your findings with the community. Remember, every debugging session, no matter how frustrating, is an opportunity to improve your skills and deepen your understanding of your codebase. Good luck, and happy debugging!
More Post